Creating Wallets
Learn how to issue and organize your organisation's wallets
Why create wallets?
Wallets provide you with the ability to compartmentalize your business' funds, allowing you to manage risk more easily.
Whenever you add funds to your organization, those funds are allocated to your business' root wallet. Similarly, whenever you issue cards, if not specified, those cards will be associated with your root wallet and draw funds from it when making purchases.
By creating separate wallets, you have more control over which cards are able to access certain funds.
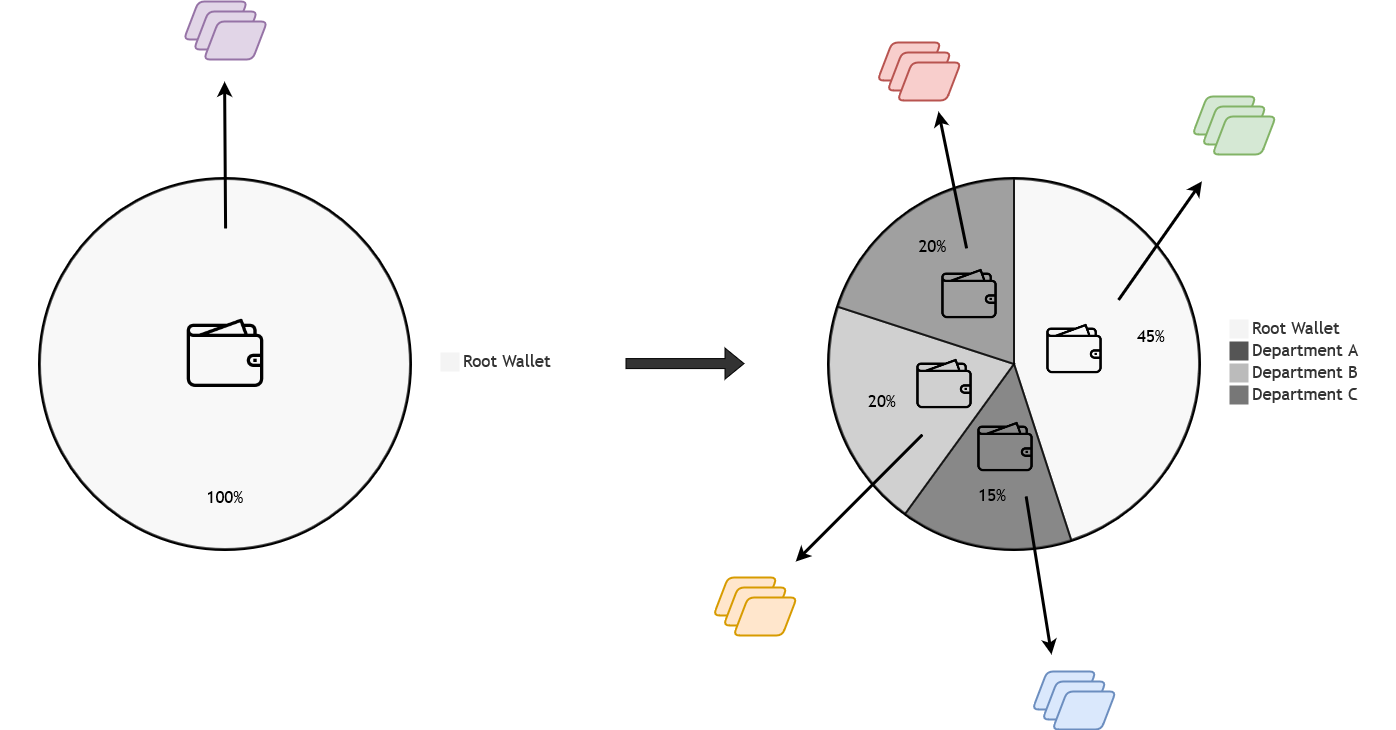
For example, if your business has multiple departments, you could create a wallet for each department, and allocate individual budgets for those departments. If you create a card for a department, you can allocate the card to that department's wallet. In this way, your department finances are separated, and have a spending capped by their wallet's funds.
Example: Create a wallet and assign it to the root wallet
import os
import requests
API_URL = "https://api.dba.thepennyinc.com/v1"
ACCESS_TOKEN = os.getenv("PENNY_ACCESS_TOKEN")
payload = {
"alias": "My New Wallet",
"balance": 500,
"overdraw_allowance": 100,
}
headers = {
"Authorization": f"Bearer {ACCESS_TOKEN}"
}
response = requests.post(API_URL + "/wallets", headers=headers, json=payload)
Example: Create a wallet and assign it to a non-root wallet
import os
import requests
API_URL = "https://api.dba.thepennyinc.com/v1"
ACCESS_TOKEN = os.getenv("PENNY_ACCESS_TOKEN")
payload = {
"alias": "My New Wallet",
"balance": 500,
"overdraw_allowance": 100,
"parent_wallet": "<id of parent wallet>
}
headers = {
"Authorization": f"Bearer {ACCESS_TOKEN}"
}
response = requests.post(API_URL + "/wallets", headers=headers, json=payload)
Updated 2 months ago